Create an Interactive HTML Report for Office 365 with PowerShell
Table of Contents
From time to time you may get clients or even managers requesting reports about their Office 365 environment. Another popular reason to compile a report on an Office 365 tenant would be when you take over a new client and you are auditing their environment trying to figure out what exactly is going on.
Click here to view the report as we go through it.
Using the PowerShell module “ReportHTML“, we can create beautiful and interactive HTML reports. Below I will walk you through a report I spent some time creating that includes tabs, charts, data tables and more. Below you can see the first page of my report. You can navigate to different content by clicking the tab. If you wanted to view the Users report you would just click on that tab.
Report Overview
Dashboard
The Dashboard contains some basic information about the Office 365 tenant including the following:
- Company information
- Global Administrators
- Strong Password Enforcement
- Recent E-Mails
- Domains
One of the greatest benefits of this report is that we can interact with the data whenever we would like. If you notice the reports “Recent E-Mails” contains a search bar. If we had more recent E-mails we can either go to the next page of results by click “Next” or we can search for a keyword which will filter the existing data.
Groups
The Groups report shows us the name of each group, the type of group, members and the E-mail address of each group. Below is an interactive char that gives us an overview of what types of groups are found in our tenant. As we see above, my tenant is made up of mostly Distribution Groups.
Licenses
The Licenses report displays each license in the tenant and their total counts, assigned count and unassigned count. Using a PowerShell HashTable we are able to convert the SkuID of the license and convert it to a much friendlier name. If the HashTable doesn’t have the SkuId it will default back to the SKU.
Below that we have two interactive charts that show total licenses by type of license and another chart that show licenses assigned by type. In this tenant, we have much more E3 licenses assigned than we do Office ProPlus.
Users
The Users report displays a wealth of information. Here you will have the Name of all your users, their UserPrincipalName, which license each user has assigned to them, the last login, If the user is disabled or not, and finally all of their alias e-mail addresses.
The data table will display the first 15 results, we can have it display 15, 25, 50, 100 or All the results by using the drop-down on the top left corner. We can filter the current data by using the search bar in the top right-hand corner.
The chart displays licensed users and unlicensed users. In my tenant, I have a little more users without a license than I do with them.
Shared Mailboxes
Shared Mailboxes will display the name of each Shared Mailbox, the primary email, and all other alias e-mail addresses.
Contacts
The Contacts report has two separate reports contained within it. The first report is the Mail Contact report which will display the name of each Mail Contact and their external e-mail address. The second report is the Mail Users report which will display the name of each Mail User, the primary e-m, il and all other alias e-mail addresses.
Resources
Similarly to the Contact report, the Resources report has two reports within it. The first one is Room Mailboxes and will display each Room Mailbox Name, Primary E-Mail, and all other alias e-mail addresses.
The second report is Equipment Mailboxes and will display each Equipment Mailbox Name, Primary E-Mail and all other alias e-mail addresses.
Features
Charts
The report contains rich interactive charts. When you hover over it you will see the values.
Filter Data
Using Data Tables we can filter the dataset to find exactly what we need. In my example below I want to see all groups that Brad Robertson is a member of. If I have a lot of groups I may not want to spend the time trying to find his name.
By just typing “Brad” I can see the only group he is a member of is a Distribution List named “Managers”. You can even see that as we start typing the data is already being filtered out before we even finish.
Ordering
By default all the data you will automatically be ordered alphabetically by name. But if you want to order it by something else you can by clicking on that property. In my example, I want to find all users that are currently locked-out or disabled. By clicking on the “Disabled” property in the user’s report I can see which users show a “True”. It will filter against all users, even though by default I am only showing the first 15 results.
Friendly License Names
By using a HashTable we can convert the AccountSKU to a much more friendly name. If the HashTable does not have a friendly name for the SKU it will fail back and use the SKU
Error Handling
When the report is gathering its data, if it comes across an empty collection (no users with strong password enforemcent disabled, no resource mailboxes, etc) it will display a friendly message in the report instead of an error in the console
2FA Support
If your global admin uses 2FA/MFA you can connect by setting the $2FA variable to $True. You will need the Exchange Online MFA Module.
How to Run the Script
If you don’t’ need to modify anything with the report at all then this will be incredibly simple to run because it only requires 2 modules and if you have Office 365, odds are you already have one of them.
Once you have the 2 modules installed, copy or download the script and you can just run it as is (but you may want to modify the first 3 lines where it specifies the left image, right image and output path). It will prompt you for Office 365 credentials so it can connect to Office 365 to extract the data.
Modules
You need two modules to run this report
Permissions
No special permissions are required. You don’t need to set up a registered app or API access. It’s best to use your tenant administrator account which is most likely the account you use anyways when managing Office 365 via PowerShell.
Etc..
You will most likely want to modify the first 3 lines of the script, these determine the left logo, right logo and the directory you save the report in. Once the script has finished running it will launch the HTML report automatically as well.
The great part of having an HTML report is when you send it to your customer or manager, they can interact with it the same way you do. There are no extra files needed, everything is embedded within the HTML file.
If you want to play more with the ReportHTML module I recommend checking out this website. It takes a little bit to learn how everything is formatted but once you grasp the formatting you will realize how easy making HTML reports using the module is.
You can find this report also on GitHub here. As I modify the report I will update this article and the GitHub repository.
The Script
<# .NOTES =========================================================================== Updated on: 6/26/2018 Created by: /u/TheLazyAdministrator Contributors: /u/jmn_lab, /u/nothingpersonalbro =========================================================================== AzureAD Module is required Install-Module -Name AzureAD https://www.powershellgallery.com/packages/azuread/ ReportHTML Moduile is required Install-Module -Name ReportHTML https://www.powershellgallery.com/packages/ReportHTML/ .DESCRIPTION Generate an interactive HTML report on your Office 365 tenant. Report on Users, Tenant information, Groups, Policies, Contacts, Mail Users, Licenses and more! .LinkCreate an Interactive HTML Report for Office 365 with PowerShell#> ######################################### # # # VARIABLES # # # ######################################### #Company logo that will be displayed on the left, can be URL or UNC $CompanyLogo = "https://www.thelazyadministrator.com/wp-content/uploads/2018/06/logo-2-e1529684959389.png" #Logo that will be on the right side, UNC or URL $RightLogo = "https://www.thelazyadministrator.com/wp-content/uploads/2018/06/amd.png" #Location the report will be saved to $ReportSavePath = "C:\Automation\" #Variable to filter licenses out, in current state will only get licenses with a count less than 9,000 this will help filter free/trial licenses $LicenseFilter = "9000" #If you want to include users last logon mailbox timestamp, set this to true $IncludeLastLogonTimestamp = $False #Set to $True if your global admin requires 2FA $2FA = $False ######################################## If ($2FA -eq $False) { $credential = Get-Credential -Message "Please enter your Office 365 credentials" Import-Module AzureAD Connect-AzureAD -Credential $credential $exchangeSession = New-PSSession -ConfigurationName Microsoft.Exchange -ConnectionUri "https://outlook.office365.com/powershell-liveid/" -Authentication "Basic" -AllowRedirection -Credential $credential Import-PSSession $exchangeSession -AllowClobber } Else { $Modules = dir $Env:LOCALAPPDATA\Apps\2.0\*\CreateExoPSSession.ps1 -Recurse | Select-Object -ExpandProperty Target -First 1 foreach ($Module in $Modules) { Import-Module "$Module" } #Connect to MSOnline w/2FA Connect-AzureAD #Connect to Exchange Online w/ 2FA Connect-EXOPSSession } $Table = New-Object 'System.Collections.Generic.List[System.Object]' $LicenseTable = New-Object 'System.Collections.Generic.List[System.Object]' $UserTable = New-Object 'System.Collections.Generic.List[System.Object]' $SharedMailboxTable = New-Object 'System.Collections.Generic.List[System.Object]' $GroupTypetable = New-Object 'System.Collections.Generic.List[System.Object]' $IsLicensedUsersTable = New-Object 'System.Collections.Generic.List[System.Object]' $ContactTable = New-Object 'System.Collections.Generic.List[System.Object]' $MailUser = New-Object 'System.Collections.Generic.List[System.Object]' $ContactMailUserTable = New-Object 'System.Collections.Generic.List[System.Object]' $RoomTable = New-Object 'System.Collections.Generic.List[System.Object]' $EquipTable = New-Object 'System.Collections.Generic.List[System.Object]' $GlobalAdminTable = New-Object 'System.Collections.Generic.List[System.Object]' $StrongPasswordTable = New-Object 'System.Collections.Generic.List[System.Object]' $CompanyInfoTable = New-Object 'System.Collections.Generic.List[System.Object]' $MessageTraceTable = New-Object 'System.Collections.Generic.List[System.Object]' $DomainTable = New-Object 'System.Collections.Generic.List[System.Object]' $Sku = @{ "O365_BUSINESS_ESSENTIALS" = "Office 365 Business Essentials" "O365_BUSINESS_PREMIUM" = "Office 365 Business Premium" "DESKLESSPACK" = "Office 365 (Plan K1)" "DESKLESSWOFFPACK" = "Office 365 (Plan K2)" "LITEPACK" = "Office 365 (Plan P1)" "EXCHANGESTANDARD" = "Office 365 Exchange Online Only" "STANDARDPACK" = "Enterprise Plan E1" "STANDARDWOFFPACK" = "Office 365 (Plan E2)" "ENTERPRISEPACK" = "Enterprise Plan E3" "ENTERPRISEPACKLRG" = "Enterprise Plan E3" "ENTERPRISEWITHSCAL" = "Enterprise Plan E4" "STANDARDPACK_STUDENT" = "Office 365 (Plan A1) for Students" "STANDARDWOFFPACKPACK_STUDENT" = "Office 365 (Plan A2) for Students" "ENTERPRISEPACK_STUDENT" = "Office 365 (Plan A3) for Students" "ENTERPRISEWITHSCAL_STUDENT" = "Office 365 (Plan A4) for Students" "STANDARDPACK_FACULTY" = "Office 365 (Plan A1) for Faculty" "STANDARDWOFFPACKPACK_FACULTY" = "Office 365 (Plan A2) for Faculty" "ENTERPRISEPACK_FACULTY" = "Office 365 (Plan A3) for Faculty" "ENTERPRISEWITHSCAL_FACULTY" = "Office 365 (Plan A4) for Faculty" "ENTERPRISEPACK_B_PILOT" = "Office 365 (Enterprise Preview)" "STANDARD_B_PILOT" = "Office 365 (Small Business Preview)" "VISIOCLIENT" = "Visio Pro Online" "POWER_BI_ADDON" = "Office 365 Power BI Addon" "POWER_BI_INDIVIDUAL_USE" = "Power BI Individual User" "POWER_BI_STANDALONE" = "Power BI Stand Alone" "POWER_BI_STANDARD" = "Power-BI Standard" "PROJECTESSENTIALS" = "Project Lite" "PROJECTCLIENT" = "Project Professional" "PROJECTONLINE_PLAN_1" = "Project Online" "PROJECTONLINE_PLAN_2" = "Project Online and PRO" "ProjectPremium" = "Project Online Premium" "ECAL_SERVICES" = "ECAL" "EMS" = "Enterprise Mobility Suite" "RIGHTSMANAGEMENT_ADHOC" = "Windows Azure Rights Management" "MCOMEETADV" = "PSTN conferencing" "SHAREPOINTSTORAGE" = "SharePoint storage" "PLANNERSTANDALONE" = "Planner Standalone" "CRMIUR" = "CMRIUR" "BI_AZURE_P1" = "Power BI Reporting and Analytics" "INTUNE_A" = "Windows Intune Plan A" "PROJECTWORKMANAGEMENT" = "Office 365 Planner Preview" "ATP_ENTERPRISE" = "Exchange Online Advanced Threat Protection" "EQUIVIO_ANALYTICS" = "Office 365 Advanced eDiscovery" "AAD_BASIC" = "Azure Active Directory Basic" "RMS_S_ENTERPRISE" = "Azure Active Directory Rights Management" "AAD_PREMIUM" = "Azure Active Directory Premium" "MFA_PREMIUM" = "Azure Multi-Factor Authentication" "STANDARDPACK_GOV" = "Microsoft Office 365 (Plan G1) for Government" "STANDARDWOFFPACK_GOV" = "Microsoft Office 365 (Plan G2) for Government" "ENTERPRISEPACK_GOV" = "Microsoft Office 365 (Plan G3) for Government" "ENTERPRISEWITHSCAL_GOV" = "Microsoft Office 365 (Plan G4) for Government" "DESKLESSPACK_GOV" = "Microsoft Office 365 (Plan K1) for Government" "ESKLESSWOFFPACK_GOV" = "Microsoft Office 365 (Plan K2) for Government" "EXCHANGESTANDARD_GOV" = "Microsoft Office 365 Exchange Online (Plan 1) only for Government" "EXCHANGEENTERPRISE_GOV" = "Microsoft Office 365 Exchange Online (Plan 2) only for Government" "SHAREPOINTDESKLESS_GOV" = "SharePoint Online Kiosk" "EXCHANGE_S_DESKLESS_GOV" = "Exchange Kiosk" "RMS_S_ENTERPRISE_GOV" = "Windows Azure Active Directory Rights Management" "OFFICESUBSCRIPTION_GOV" = "Office ProPlus" "MCOSTANDARD_GOV" = "Lync Plan 2G" "SHAREPOINTWAC_GOV" = "Office Online for Government" "SHAREPOINTENTERPRISE_GOV" = "SharePoint Plan 2G" "EXCHANGE_S_ENTERPRISE_GOV" = "Exchange Plan 2G" "EXCHANGE_S_ARCHIVE_ADDON_GOV" = "Exchange Online Archiving" "EXCHANGE_S_DESKLESS" = "Exchange Online Kiosk" "SHAREPOINTDESKLESS" = "SharePoint Online Kiosk" "SHAREPOINTWAC" = "Office Online" "YAMMER_ENTERPRISE" = "Yammer for the Starship Enterprise" "EXCHANGE_L_STANDARD" = "Exchange Online (Plan 1)" "MCOLITE" = "Lync Online (Plan 1)" "SHAREPOINTLITE" = "SharePoint Online (Plan 1)" "OFFICE_PRO_PLUS_SUBSCRIPTION_SMBIZ" = "Office ProPlus" "EXCHANGE_S_STANDARD_MIDMARKET" = "Exchange Online (Plan 1)" "MCOSTANDARD_MIDMARKET" = "Lync Online (Plan 1)" "SHAREPOINTENTERPRISE_MIDMARKET" = "SharePoint Online (Plan 1)" "OFFICESUBSCRIPTION" = "Office ProPlus" "YAMMER_MIDSIZE" = "Yammer" "DYN365_ENTERPRISE_PLAN1" = "Dynamics 365 Customer Engagement Plan Enterprise Edition" "ENTERPRISEPREMIUM_NOPSTNCONF" = "Enterprise E5 (without Audio Conferencing)" "ENTERPRISEPREMIUM" = "Enterprise E5 (with Audio Conferencing)" "MCOSTANDARD" = "Skype for Business Online Standalone Plan 2" "PROJECT_MADEIRA_PREVIEW_IW_SKU" = "Dynamics 365 for Financials for IWs" "STANDARDWOFFPACK_IW_STUDENT" = "Office 365 Education for Students" "STANDARDWOFFPACK_IW_FACULTY" = "Office 365 Education for Faculty" "EOP_ENTERPRISE_FACULTY" = "Exchange Online Protection for Faculty" "EXCHANGESTANDARD_STUDENT" = "Exchange Online (Plan 1) for Students" "OFFICESUBSCRIPTION_STUDENT" = "Office ProPlus Student Benefit" "STANDARDWOFFPACK_FACULTY" = "Office 365 Education E1 for Faculty" "STANDARDWOFFPACK_STUDENT" = "Microsoft Office 365 (Plan A2) for Students" "DYN365_FINANCIALS_BUSINESS_SKU" = "Dynamics 365 for Financials Business Edition" "DYN365_FINANCIALS_TEAM_MEMBERS_SKU" = "Dynamics 365 for Team Members Business Edition" "FLOW_FREE" = "Microsoft Flow Free" "POWER_BI_PRO" = "Power BI Pro" "O365_BUSINESS" = "Office 365 Business" "DYN365_ENTERPRISE_SALES" = "Dynamics Office 365 Enterprise Sales" "RIGHTSMANAGEMENT" = "Rights Management" "PROJECTPROFESSIONAL" = "Project Professional" "VISIOONLINE_PLAN1" = "Visio Online Plan 1" "EXCHANGEENTERPRISE" = "Exchange Online Plan 2" "DYN365_ENTERPRISE_P1_IW" = "Dynamics 365 P1 Trial for Information Workers" "DYN365_ENTERPRISE_TEAM_MEMBERS" = "Dynamics 365 For Team Members Enterprise Edition" "CRMSTANDARD" = "Microsoft Dynamics CRM Online Professional" "EXCHANGEARCHIVE_ADDON" = "Exchange Online Archiving For Exchange Online" "EXCHANGEDESKLESS" = "Exchange Online Kiosk" "SPZA_IW" = "App Connect" "WINDOWS_STORE" = "Windows Store for Business" "MCOEV" = "Microsoft Phone System" "VIDEO_INTEROP" = "Polycom Skype Meeting Video Interop for Skype for Business" "SPE_E5" = "Microsoft 365 E5" "SPE_E3" = "Microsoft 365 E3" "ATA" = "Advanced Threat Analytics" "MCOPSTN2" = "Domestic and International Calling Plan" "FLOW_P1" = "Microsoft Flow Plan 1" "FLOW_P2" = "Microsoft Flow Plan 2" } # Get all users right away. Instead of doing several lookups, we will use this object to look up all the information needed. $AllUsers = get-azureaduser -All:$true #Company Information $CompanyInfo = Get-AzureADTenantDetail $CompanyName = $CompanyInfo.DisplayName $TechEmail = $CompanyInfo.TechnicalNotificationMails | Out-String $DirSync = $CompanyInfo.DirSyncEnabled $LastDirSync = $CompanyInfo.CompanyLastDirSyncTime If ($DirSync -eq $Null) { $LastDirSync = "Not Available" $DirSync = "Disabled" } If ($PasswordSync -eq $Null) { $LastPasswordSync = "Not Available" } $obj = [PSCustomObject]@{ 'Name' = $CompanyName 'Technical E-mail' = $TechEmail 'Directory Sync' = $DirSync 'Last Directory Sync' = $LastDirSync } $CompanyInfoTable.add($obj) #Get Tenant Global Admins $role = Get-AzureADDirectoryRole | Where-Object { $_.DisplayName -match "Company Administrator" } $Admins = Get-AzureADDirectoryRoleMember -ObjectId $role.ObjectId Foreach ($Admin in $Admins) { $Name = $Admin.DisplayName $EmailAddress = $Admin.Mail if (($admin.assignedlicenses.SkuID) -ne $Null) { $Licensed = $True } else { $Licensed = $False } $obj = [PSCustomObject]@{ 'Name' = $Name 'Is Licensed' = $Licensed 'E-Mail Address' = $EmailAddress } $GlobalAdminTable.add($obj) } #Users with Strong Password Disabled $LooseUsers = $AllUsers | Where-Object { $_.PasswordPolicies -eq "DisableStrongPassword" } Foreach ($LooseUser in $LooseUsers) { $NameLoose = $LooseUser.DisplayName $UPNLoose = $LooseUser.UserPrincipalName $StrongPasswordLoose = "False" if (($LooseUser.assignedlicenses.SkuID) -ne $Null) { $LicensedLoose = $true } else { $LicensedLoose = $false } $obj = [PSCustomObject]@{ 'Name' = $NameLoose 'UserPrincipalName' = $UPNLoose 'Is Licensed' = $LicensedLoose 'Strong Password Required' = $StrongPasswordLoose } $StrongPasswordTable.add($obj) } If (($StrongPasswordTable).count -eq 0) { $StrongPasswordTable = [PSCustomObject]@{ 'Information' = 'Information: No Users were found with Strong Password Enforcement disabled' } } #Message Trace / Recent Messages $RecentMessages = Get-MessageTrace Foreach ($RecentMessage in $RecentMessages) { $TraceDate = $RecentMessage.Received $Sender = $RecentMessage.SenderAddress $Recipient = $RecentMessage.RecipientAddress $Subject = $RecentMessage.Subject $Status = $RecentMessage.Status $obj = [PSCustomObject]@{ 'Received Date' = $TraceDate 'E-Mail Subject' = $Subject 'Sender' = $Sender 'Recipient' = $Recipient 'Status' = $Status } $MessageTraceTable.add($obj) } If (($MessageTraceTable).count -eq 0) { $MessageTraceTable = [PSCustomObject]@{ 'Information' = 'Information: No recent E-Mails were found' } } #Tenant Domain $Domains = Get-AzureAdDomain foreach ($Domain in $Domains) { $DomainName = $Domain.Name $Verified = $Domain.IsVerified $DefaultStatus = $Domain.IsDefault $obj = [PSCustomObject]@{ 'Domain Name' = $DomainName 'Verification Status' = $Verified 'Default' = $DefaultStatus } $DomainTable.add($obj) } #Get groups and sort in alphabetical order $Groups = Get-AzureAdGroup -All $True | Sort-Object DisplayName $DistroCount = ($Groups | Where-Object { $_.MailEnabled -eq $true -and $_.SecurityEnabled -eq $false }).Count $obj1 = [PSCustomObject]@{ 'Name' = 'Distribution Group' 'Count' = $DistroCount } $GroupTypetable.add($obj1) $SecurityCount = ($Groups | Where-Object { $_.MailEnabled -eq $false -and $_.SecurityEnabled -eq $true }).Count $obj1 = [PSCustomObject]@{ 'Name' = 'Security Group' 'Count' = $SecurityCount } $GroupTypetable.add($obj1) $SecurityMailEnabledCount = ($Groups | Where-Object { $_.MailEnabled -eq $true -and $_.SecurityEnabled -eq $true }).Count $obj1 = [PSCustomObject]@{ 'Name' = 'Mail Enabled Security Group' 'Count' = $SecurityMailEnabledCount } $GroupTypetable.add($obj1) Foreach ($Group in $Groups) { $Type = New-Object 'System.Collections.Generic.List[System.Object]' if ($group.MailEnabled -eq $True -and $group.SecurityEnabled -eq $False) { $Type = "Distribution Group" } if ($group.MailEnabled -eq $False -and $group.SecurityEnabled -eq $True) { $Type = "Security Group" } if ($group.MailEnabled -eq $True -and $group.SecurityEnabled -eq $True) { $Type = "Mail Enabled Security Group" } $Users = (Get-AzureADGroupMember -ObjectId $Group.ObjectID | Sort-Object DisplayName | Select-Object -ExpandProperty DisplayName) -join ", " $GName = $Group.DisplayName $hash = New-Object PSObject -property @{ Name = "$GName"; Type = "$Type"; Members = "$Users" } $GEmail = $Group.Mail $obj = [PSCustomObject]@{ 'Name' = $GName 'Type' = $Type 'Members' = $users 'E-mail Address' = $GEmail } $table.add($obj) } If (($table).count -eq 0) { $table = [PSCustomObject]@{ 'Information' = 'Information: No Groups were found in the tenant' } } #Get all licenses $Licenses = Get-AzureADSubscribedSku #Split licenses at colon Foreach ($License in $Licenses) { $TextLic = $null $ASku = ($License).SkuPartNumber $TextLic = $Sku.Item("$ASku") If (!($TextLic)) { $OLicense = $License.SkuPartNumber } Else { $OLicense = $TextLic } $TotalAmount = $License.PrepaidUnits.enabled $Assigned = $License.ConsumedUnits $Unassigned = ($TotalAmount - $Assigned) If ($TotalAmount -lt $LicenseFilter) { $obj = [PSCustomObject]@{ 'Name' = $Olicense 'Total Amount' = $TotalAmount 'Assigned Licenses' = $Assigned 'Unassigned Licenses' = $Unassigned } $licensetable.add($obj) } } If (($licensetable).count -eq 0) { $licensetable = [PSCustomObject]@{ 'Information' = 'Information: No Licenses were found in the tenant' } } $IsLicensed = ($AllUsers | Where-Object { $_.assignedlicenses.count -gt 0 }).Count $objULic = [PSCustomObject]@{ 'Name' = 'Users Licensed' 'Count' = $IsLicensed } $IsLicensedUsersTable.add($objULic) $ISNotLicensed = ($AllUsers | Where-Object { $_.assignedlicenses.count -eq 0 }).Count $objULic = [PSCustomObject]@{ 'Name' = 'Users Not Licensed' 'Count' = $IsNotLicensed } $IsLicensedUsersTable.add($objULic) If (($IsLicensedUsersTable).count -eq 0) { $IsLicensedUsersTable = [PSCustomObject]@{ 'Information' = 'Information: No Licenses were found in the tenant' } } Foreach ($User in $AllUsers) { $ProxyA = New-Object 'System.Collections.Generic.List[System.Object]' $NewObject02 = New-Object 'System.Collections.Generic.List[System.Object]' $NewObject01 = New-Object 'System.Collections.Generic.List[System.Object]' $UserLicenses = ($user | Select -ExpandProperty AssignedLicenses).SkuID If (($UserLicenses).count -gt 1) { Foreach ($UserLicense in $UserLicenses) { $UserLicense = ($licenses | Where-Object { $_.skuid -match $UserLicense }).SkuPartNumber $TextLic = $Sku.Item("$UserLicense") If (!($TextLic)) { $NewObject01 = [PSCustomObject]@{ 'Licenses' = $UserLicense } $NewObject02.add($NewObject01) } Else { $NewObject01 = [PSCustomObject]@{ 'Licenses' = $textlic } $NewObject02.add($NewObject01) } } } Elseif (($UserLicenses).count -eq 1) { $lic = ($licenses | Where-Object { $_.skuid -match $UserLicenses}).SkuPartNumber $TextLic = $Sku.Item("$lic") If (!($TextLic)) { $NewObject01 = [PSCustomObject]@{ 'Licenses' = $lic } $NewObject02.add($NewObject01) } Else { $NewObject01 = [PSCustomObject]@{ 'Licenses' = $textlic } $NewObject02.add($NewObject01) } } Else { $NewObject01 = [PSCustomObject]@{ 'Licenses' = $Null } $NewObject02.add($NewObject01) } $ProxyAddresses = ($User | Select-Object -ExpandProperty ProxyAddresses) If ($ProxyAddresses -ne $Null) { Foreach ($Proxy in $ProxyAddresses) { $ProxyB = $Proxy -split ":" | Select-Object -Last 1 $ProxyA.add($ProxyB) } $ProxyC = $ProxyA -join ", " } Else { $ProxyC = $Null } $Name = $User.DisplayName $UPN = $User.UserPrincipalName $UserLicenses = ($NewObject02 | Select-Object -ExpandProperty Licenses) -join ", " $Enabled = $User.AccountEnabled $ResetPW = Get-User $User.DisplayName | Select-Object -ExpandProperty ResetPasswordOnNextLogon If ($IncludeLastLogonTimestamp -eq $True) { $LastLogon = Get-Mailbox $User.DisplayName | Get-MailboxStatistics -ErrorAction SilentlyContinue | Select-Object -ExpandProperty LastLogonTime -ErrorAction SilentlyContinue $obj = [PSCustomObject]@{ 'Name' = $Name 'UserPrincipalName' = $UPN 'Licenses' = $UserLicenses 'Last Mailbox Logon' = $LastLogon 'Reset Password at Next Logon' = $ResetPW 'Enabled' = $Enabled 'E-mail Addresses' = $ProxyC } } Else { $obj = [PSCustomObject]@{ 'Name' = $Name 'UserPrincipalName' = $UPN 'Licenses' = $UserLicenses 'Reset Password at Next Logon' = $ResetPW 'Enabled' = $Enabled 'E-mail Addresses' = $ProxyC } } $usertable.add($obj) } If (($usertable).count -eq 0) { $usertable = [PSCustomObject]@{ 'Information' = 'Information: No Users were found in the tenant' } } #Get all Shared Mailboxes $SharedMailboxes = Get-Recipient -Resultsize unlimited | Where-Object { $_.RecipientTypeDetails -eq "SharedMailbox" } Foreach ($SharedMailbox in $SharedMailboxes) { $ProxyA = New-Object 'System.Collections.Generic.List[System.Object]' $Name = $SharedMailbox.Name $PrimEmail = $SharedMailbox.PrimarySmtpAddress $ProxyAddresses = ($SharedMailbox | Where-Object { $_.EmailAddresses -notlike "*$PrimEmail*" } | Select-Object -ExpandProperty EmailAddresses) If ($ProxyAddresses -ne $Null) { Foreach ($ProxyAddress in $ProxyAddresses) { $ProxyB = $ProxyAddress -split ":" | Select-Object -Last 1 If ($ProxyB -eq $PrimEmail) { $ProxyB = $Null } $ProxyA.add($ProxyB) $ProxyC = $ProxyA } } Else { $ProxyC = $Null } $ProxyF = ($ProxyC -join ", ").TrimEnd(", ") $obj = [PSCustomObject]@{ 'Name' = $Name 'Primary E-Mail' = $PrimEmail 'E-mail Addresses' = $ProxyF } $SharedMailboxTable.add($obj) } If (($SharedMailboxTable).count -eq 0) { $SharedMailboxTable = [PSCustomObject]@{ 'Information' = 'Information: No Shared Mailboxes were found in the tenant' } } #Get all Contacts $Contacts = Get-MailContact #Split licenses at colon Foreach ($Contact in $Contacts) { $ContactName = $Contact.DisplayName $ContactPrimEmail = $Contact.PrimarySmtpAddress $objContact = [PSCustomObject]@{ 'Name' = $ContactName 'E-mail Address' = $ContactPrimEmail } $ContactTable.add($objContact) } If (($ContactTable).count -eq 0) { $ContactTable = [PSCustomObject]@{ 'Information' = 'Information: No Contacts were found in the tenant' } } #Get all Mail Users $MailUsers = Get-MailUser foreach ($MailUser in $mailUsers) { $MailArray = New-Object 'System.Collections.Generic.List[System.Object]' $MailPrimEmail = $MailUser.PrimarySmtpAddress $MailName = $MailUser.DisplayName $MailEmailAddresses = ($MailUser.EmailAddresses | Where-Object { $_ -cnotmatch '^SMTP' }) foreach ($MailEmailAddress in $MailEmailAddresses) { $MailEmailAddressSplit = $MailEmailAddress -split ":" | Select-Object -Last 1 $MailArray.add($MailEmailAddressSplit) } $UserEmails = $MailArray -join ", " $obj = [PSCustomObject]@{ 'Name' = $MailName 'Primary E-Mail' = $MailPrimEmail 'E-mail Addresses' = $UserEmails } $ContactMailUserTable.add($obj) } If (($ContactMailUserTable).count -eq 0) { $ContactMailUserTable = [PSCustomObject]@{ 'Information' = 'Information: No Mail Users were found in the tenant' } } $Rooms = Get-Mailbox -ResultSize Unlimited -Filter '(RecipientTypeDetails -eq "RoomMailBox")' Foreach ($Room in $Rooms) { $RoomArray = New-Object 'System.Collections.Generic.List[System.Object]' $RoomName = $Room.DisplayName $RoomPrimEmail = $Room.PrimarySmtpAddress $RoomEmails = ($Room.EmailAddresses | Where-Object { $_ -cnotmatch '^SMTP' }) foreach ($RoomEmail in $RoomEmails) { $RoomEmailSplit = $RoomEmail -split ":" | Select-Object -Last 1 $RoomArray.add($RoomEmailSplit) } $RoomEMailsF = $RoomArray -join ", " $obj = [PSCustomObject]@{ 'Name' = $RoomName 'Primary E-Mail' = $RoomPrimEmail 'E-mail Addresses' = $RoomEmailsF } $RoomTable.add($obj) } If (($RoomTable).count -eq 0) { $RoomTable = [PSCustomObject]@{ 'Information' = 'Information: No Room Mailboxes were found in the tenant' } } $EquipMailboxes = Get-Mailbox -ResultSize Unlimited -Filter '(RecipientTypeDetails -eq "EquipmentMailBox")' Foreach ($EquipMailbox in $EquipMailboxes) { $EquipArray = New-Object 'System.Collections.Generic.List[System.Object]' $EquipName = $EquipMailbox.DisplayName $EquipPrimEmail = $EquipMailbox.PrimarySmtpAddress $EquipEmails = ($EquipMailbox.EmailAddresses | Where-Object { $_ -cnotmatch '^SMTP' }) foreach ($EquipEmail in $EquipEmails) { $EquipEmailSplit = $EquipEmail -split ":" | Select-Object -Last 1 $EquipArray.add($EquipEmailSplit) } $EquipEMailsF = $EquipArray -join ", " $obj = [PSCustomObject]@{ 'Name' = $EquipName 'Primary E-Mail' = $EquipPrimEmail 'E-mail Addresses' = $EquipEmailsF } $EquipTable.add($obj) } If (($EquipTable).count -eq 0) { $EquipTable = [PSCustomObject]@{ 'Information' = 'Information: No Equipment Mailboxes were found in the tenant' } } $tabarray = @('Dashboard','Groups', 'Licenses', 'Users', 'Shared Mailboxes', 'Contacts', 'Resources') #basic Properties $PieObject2 = Get-HTMLPieChartObject $PieObject2.Title = "Office 365 Total Licenses" $PieObject2.Size.Height = 250 $PieObject2.Size.width = 250 $PieObject2.ChartStyle.ChartType = 'doughnut' #These file exist in the module directoy, There are 4 schemes by default $PieObject2.ChartStyle.ColorSchemeName = "ColorScheme4" #There are 8 generated schemes, randomly generated at runtime $PieObject2.ChartStyle.ColorSchemeName = "Generated7" #you can also ask for a random scheme. Which also happens if you have too many records for the scheme $PieObject2.ChartStyle.ColorSchemeName = 'Random' #Data defintion you can reference any column from name and value from the dataset. #Name and Count are the default to work with the Group function. $PieObject2.DataDefinition.DataNameColumnName = 'Name' $PieObject2.DataDefinition.DataValueColumnName = 'Total Amount' #basic Properties $PieObject3 = Get-HTMLPieChartObject $PieObject3.Title = "Office 365 Assigned Licenses" $PieObject3.Size.Height = 250 $PieObject3.Size.width = 250 $PieObject3.ChartStyle.ChartType = 'doughnut' #These file exist in the module directoy, There are 4 schemes by default $PieObject3.ChartStyle.ColorSchemeName = "ColorScheme4" #There are 8 generated schemes, randomly generated at runtime $PieObject3.ChartStyle.ColorSchemeName = "Generated5" #you can also ask for a random scheme. Which also happens if you have too many records for the scheme $PieObject3.ChartStyle.ColorSchemeName = 'Random' #Data defintion you can reference any column from name and value from the dataset. #Name and Count are the default to work with the Group function. $PieObject3.DataDefinition.DataNameColumnName = 'Name' $PieObject3.DataDefinition.DataValueColumnName = 'Assigned Licenses' #basic Properties $PieObject4 = Get-HTMLPieChartObject $PieObject4.Title = "Office 365 Unassigned Licenses" $PieObject4.Size.Height = 250 $PieObject4.Size.width = 250 $PieObject4.ChartStyle.ChartType = 'doughnut' #These file exist in the module directoy, There are 4 schemes by default $PieObject4.ChartStyle.ColorSchemeName = "ColorScheme4" #There are 8 generated schemes, randomly generated at runtime $PieObject4.ChartStyle.ColorSchemeName = "Generated4" #you can also ask for a random scheme. Which also happens if you have too many records for the scheme $PieObject4.ChartStyle.ColorSchemeName = 'Random' #Data defintion you can reference any column from name and value from the dataset. #Name and Count are the default to work with the Group function. $PieObject4.DataDefinition.DataNameColumnName = 'Name' $PieObject4.DataDefinition.DataValueColumnName = 'Unassigned Licenses' #basic Properties $PieObjectGroupType = Get-HTMLPieChartObject $PieObjectGroupType.Title = "Office 365 Groups" $PieObjectGroupType.Size.Height = 250 $PieObjectGroupType.Size.width = 250 $PieObjectGroupType.ChartStyle.ChartType = 'doughnut' #These file exist in the module directoy, There are 4 schemes by default $PieObjectGroupType.ChartStyle.ColorSchemeName = "ColorScheme4" #There are 8 generated schemes, randomly generated at runtime $PieObjectGroupType.ChartStyle.ColorSchemeName = "Generated8" #you can also ask for a random scheme. Which also happens if you have too many records for the scheme $PieObjectGroupType.ChartStyle.ColorSchemeName = 'Random' #Data defintion you can reference any column from name and value from the dataset. #Name and Count are the default to work with the Group function. $PieObjectGroupType.DataDefinition.DataNameColumnName = 'Name' $PieObjectGroupType.DataDefinition.DataValueColumnName = 'Count' ##--LICENSED AND UNLICENSED USERS PIE CHART--## #basic Properties $PieObjectULicense = Get-HTMLPieChartObject $PieObjectULicense.Title = "License Status" $PieObjectULicense.Size.Height = 250 $PieObjectULicense.Size.width = 250 $PieObjectULicense.ChartStyle.ChartType = 'doughnut' #These file exist in the module directoy, There are 4 schemes by default $PieObjectULicense.ChartStyle.ColorSchemeName = "ColorScheme3" #There are 8 generated schemes, randomly generated at runtime $PieObjectULicense.ChartStyle.ColorSchemeName = "Generated3" #you can also ask for a random scheme. Which also happens if you have too many records for the scheme $PieObjectULicense.ChartStyle.ColorSchemeName = 'Random' #Data defintion you can reference any column from name and value from the dataset. #Name and Count are the default to work with the Group function. $PieObjectULicense.DataDefinition.DataNameColumnName = 'Name' $PieObjectULicense.DataDefinition.DataValueColumnName = 'Count' $rpt = New-Object 'System.Collections.Generic.List[System.Object]' $rpt += get-htmlopenpage -TitleText 'Office 365 Tenant Report' -LeftLogoString $CompanyLogo -RightLogoString $RightLogo $rpt += Get-HTMLTabHeader -TabNames $tabarray $rpt += get-htmltabcontentopen -TabName $tabarray[0] -TabHeading ("Report: " + (Get-Date -Format MM-dd-yyyy)) $rpt+= Get-HtmlContentOpen -HeaderText "Office 365 Dashboard" $rpt += Get-HTMLContentOpen -HeaderText "Company Information" $rpt += Get-HtmlContentTable $CompanyInfoTable $rpt += Get-HTMLContentClose $rpt+= get-HtmlColumn1of2 $rpt+= Get-HtmlContentOpen -BackgroundShade 1 -HeaderText 'Global Administrators' $rpt+= get-htmlcontentdatatable $GlobalAdminTable -HideFooter $rpt+= Get-HtmlContentClose $rpt+= get-htmlColumnClose $rpt+= get-htmlColumn2of2 $rpt+= Get-HtmlContentOpen -HeaderText 'Users With Strong Password Enforcement Disabled' $rpt+= get-htmlcontentdatatable $StrongPasswordTable -HideFooter $rpt+= Get-HtmlContentClose $rpt+= get-htmlColumnClose $rpt += Get-HTMLContentOpen -HeaderText "Recent E-Mails" $rpt += Get-HTMLContentDataTable $MessageTraceTable -HideFooter $rpt += Get-HTMLContentClose $rpt += Get-HTMLContentOpen -HeaderText "Domains" $rpt += Get-HtmlContentTable $DomainTable $rpt += Get-HTMLContentClose $rpt+= Get-HtmlContentClose $rpt += get-htmltabcontentclose $rpt += get-htmltabcontentopen -TabName $tabarray[1] -TabHeading ("Report: " + (Get-Date -Format MM-dd-yyyy)) $rpt += Get-HTMLContentOpen -HeaderText "Office 365 Groups" $rpt += get-htmlcontentdatatable $Table -HideFooter $rpt += Get-HTMLContentClose $rpt += Get-HTMLContentOpen -HeaderText "Office 365 Groups Chart" $rpt += Get-HTMLPieChart -ChartObject $PieObjectGroupType -DataSet $GroupTypetable $rpt += Get-HTMLContentClose $rpt += get-htmltabcontentclose $rpt += get-htmltabcontentopen -TabName $tabarray[2] -TabHeading ("Report: " + (Get-Date -Format MM-dd-yyyy)) $rpt += Get-HTMLContentOpen -HeaderText "Office 365 Licenses" $rpt += get-htmlcontentdatatable $LicenseTable -HideFooter $rpt += Get-HTMLContentClose $rpt += Get-HTMLContentOpen -HeaderText "Office 365 Licensing Charts" $rpt += Get-HTMLColumnOpen -ColumnNumber 1 -ColumnCount 2 $rpt += Get-HTMLPieChart -ChartObject $PieObject2 -DataSet $licensetable $rpt += Get-HTMLColumnClose $rpt += Get-HTMLColumnOpen -ColumnNumber 2 -ColumnCount 2 $rpt += Get-HTMLPieChart -ChartObject $PieObject3 -DataSet $licensetable $rpt += Get-HTMLColumnClose $rpt += Get-HTMLContentclose $rpt += get-htmltabcontentclose $rpt += get-htmltabcontentopen -TabName $tabarray[3] -TabHeading ("Report: " + (Get-Date -Format MM-dd-yyyy)) $rpt += Get-HTMLContentOpen -HeaderText "Office 365 Users" $rpt += get-htmlcontentdatatable $UserTable -HideFooter $rpt += Get-HTMLContentClose $rpt += Get-HTMLContentOpen -HeaderText "Licensed & Unlicensed Users Chart" $rpt += Get-HTMLPieChart -ChartObject $PieObjectULicense -DataSet $IsLicensedUsersTable $rpt += Get-HTMLContentClose $rpt += get-htmltabcontentclose $rpt += get-htmltabcontentopen -TabName $tabarray[4] -TabHeading ("Report: " + (Get-Date -Format MM-dd-yyyy)) $rpt += Get-HTMLContentOpen -HeaderText "Office 365 Shared Mailboxes" $rpt += get-htmlcontentdatatable $SharedMailboxTable -HideFooter $rpt += Get-HTMLContentClose $rpt += get-htmltabcontentclose $rpt += get-htmltabcontentopen -TabName $tabarray[5] -TabHeading ("Report: " + (Get-Date -Format MM-dd-yyyy)) $rpt += Get-HTMLContentOpen -HeaderText "Office 365 Contacts" $rpt += get-htmlcontentdatatable $ContactTable -HideFooter $rpt += Get-HTMLContentClose $rpt += Get-HTMLContentOpen -HeaderText "Office 365 Mail Users" $rpt += get-htmlcontentdatatable $ContactMailUserTable -HideFooter $rpt += Get-HTMLContentClose $rpt += get-htmltabcontentclose $rpt += get-htmltabcontentopen -TabName $tabarray[6] -TabHeading ("Report: " + (Get-Date -Format MM-dd-yyyy)) $rpt += Get-HTMLContentOpen -HeaderText "Office 365 Room Mailboxes" $rpt += get-htmlcontentdatatable $RoomTable -HideFooter $rpt += Get-HTMLContentClose $rpt += Get-HTMLContentOpen -HeaderText "Office 365 Equipment Mailboxes" $rpt += get-htmlcontentdatatable $EquipTable -HideFooter $rpt += Get-HTMLContentClose $rpt += get-htmltabcontentclose $rpt += Get-HTMLClosePage $Day = (Get-Date).Day $Month = (Get-Date).Month $Year = (Get-Date).Year $ReportName = ("$Day" + "-" + "$Month" + "-" + "$Year" + "-" + "O365 Tenant Report") Save-HTMLReport -ReportContent $rpt -ShowReport -ReportName $ReportName -ReportPath $ReportSavePath
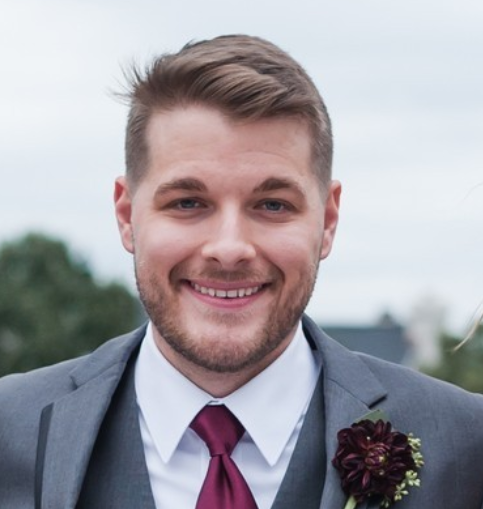
My name is Bradley Wyatt; I am a 5x Microsoft Most Valuable Professional (MVP) in Microsoft Azure and Microsoft 365. I have given talks at many different conferences, user groups, and companies throughout the United States, ranging from PowerShell to DevOps Security best practices, and I am the 2022 North American Outstanding Contribution to the Microsoft Community winner.
39 thoughts on “Create an Interactive HTML Report for Office 365 with PowerShell”
This is awesome, thank you so much for sharing this script.
Fantastic work! Any way to report on if the user has multi-factor authentication enabled? Or pull the tenant’s security score?
Hi Adam, I added this as a feature request to the GitHub project. https://github.com/bwya77/Office365Dashboard/issues/1
Hi,
I receive errors like this for every single user, any ideas?
The operation couldn’t be performed because object ‘Firstname Lastname’ couldn’t be found on ‘HE1PR10A003DC05.EURPR10A003.PROD.OUTLOOK.COM’.
+ CategoryInfo : NotSpecified: (:) [Get-Mailbox], ManagementObjectNotFoundException
+ FullyQualifiedErrorId : [Server=AM6PR10MB1927,RequestId=8e4a98eb-c2dd-4142-a624-d4361783d137,TimeStamp=2018-07-11 10:11:14] [FailureCategory=Cmdlet-ManagementObjectNotFoundException] 779D7F09,Micr
osoft.Exchange.Management.RecipientTasks.GetMailbox
+ PSComputerName : outlook.office365.com
Hello – I sent you an e-mail to gather more information
I’m getting the same error.
If you can e-mail me at Brad@TheLazyAdministrator I can work on this problem, I need some more information to get to the bottom of the issue
Works flawlessly!
If I don’t want the recent messages do I just remove that section from the script?
You would want to remove lines 296-321 where it gets recent messages, and then lines 875-877 where we add the data to an HTML Content Data Table
Hey Brad, I added a ton of SfB functionality now – was going to update the description to reflect that and then submit a pull request, but for the sample report I was either going to remove that since I don’t have a good place to put one with the new SfB info in it, or see if you wanted to update yours so I could leave the link.
Make a pull request and I can look over the changes and see what I can do!
Maybe we can add commands for send report on mail ??
Love your test users, lol.
You sir are a life saver. one question though, what would i need to add to get the report to show all members of each shared mailbox?
Can you use this script if your using MFA in your Admin Portal?
Yes set the $2FA variable to true
Are the two modules installed on the server side or client side? I’m getting ‘is not recognized as the name of a cmdlet’ error on almost every line. Do i need to have admin rights to O365 for this to work? Thanks
The modules are needed on the machine from which you run the script from.
What do I do if I’m getting ‘The term ‘example term’ is not recognized as the name of a cmdlet’ for every line?
Try and get the code again and re-run, I pushed some updates to it. https://github.com/bwya77/PSHTML-AD-Report
If you still have issues please either make a bug request in GitHub or email me at [email protected], screenshots will greatly help too if you can
Added a progress bar and it works like a champ.
I was curious if you have had any questions on timeouts when running against a large environment? I was able to run against my smaller tenant, but against the larger we timed out powershell after a while. I commented out all groups and shared mailboxes and still timed out.
try breaking the script up a bit if its timing out. or if you get an error regarding PSMemory you can modify it.
Hello,
I was just wondering how long this should take to run? I left it for about half an hour, but didn’t seem to do anything after the tennant information came up.
This message came up though..
WARNING: The names of some imported commands from the module ‘tmp_4deze4ag.z1e’ include unapproved verbs that might
make them less discoverable. To find the commands with unapproved verbs, run the Import-Module command again with the
Verbose parameter. For a list of approved verbs, type Get-Verb.
Should i just wait for it ?
Thanks
It can take a while depending on the environment. I have it as a very early morning scheduled task (500-700 users) and it takes about 15-20min. It is grabbing a lot of data and then makes the entire HTML report. You can comment items out you prefer not to report on to speed it up a bit
Hi Brad,
Thank you so much for a great script.
I do have an issue with local logos (as the ones I can find for my customers would need to be slightly modified). When I try to put a local path instead of a URL, the logos won’t load. I did open an issue about this in Github.
Any tips on how to use local logos instead of URL ones ?
This is on the ReportHTML module. If a local path does not work have you tried UNC?
I want to Learn to create report using PowerShell.
Can you refer any book?
there aren’t any books specific on PowerShell Reporting that I am aware of and can recommend. Since its just PowerShell concepts and syntax I would recommend PowerShell in a month of lunches if you have not read that
Hi Brad, You did really great work. I got few issues with this script and just wondering if you can assist me with this. Below are the errors that I am getting and it is not saving any report either. I have grabbed finalreport variable and write it to a html file but it doesn’t have any charts and also formatting looks weird too.
Cannot resolve the manager, Administrator on the group CustomerService
Done!
Working on Organizational Units Report…
Done!
Working on Users Report…
Done!
Working on Group Policy Report…
Done!
Working on Computers Report…
Get-ADComputer : The server has returned the following error: invalid enumeration context.
At D:\Scripts\PowerShell\LazyAdminAdReport.ps1:1211 char:14
+ $Computers = Get-ADComputer -Filter * -Properties *
+ ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
+ CategoryInfo : NotSpecified: (:) [Get-ADComputer], ADException
+ FullyQualifiedErrorId : ActiveDirectoryServer:0,Microsoft.ActiveDirectory.Management.Commands.GetADComputer
Done!
Compiling Report…
Get-HTMLPieChart : Cannot bind argument to parameter ‘DataSet’ because it is an empty collection.
At D:\Scripts\PowerShell\LazyAdminAdReport.ps1:1797 char:83
+ … hart -ChartObject $PieObjectComputerObjOS -DataSet $GraphComputerOS))
+ ~~~~~~~~~~~~~~~~
+ CategoryInfo : InvalidData: (:) [Get-HTMLPieChart], ParameterBindingValidationException
+ FullyQualifiedErrorId : ParameterArgumentValidationErrorEmptyCollectionNotAllowed,Get-HTMLPieChart
Save-HTMLReport : Cannot bind argument to parameter ‘ReportContent’ because it is null.
At D:\Scripts\PowerShell\LazyAdminAdReport.ps1:1808 char:32
+ Save-HTMLReport -ReportContent $FinalReport -ShowReport -ReportName $ …
+ ~~~~~~~~~~~~
+ CategoryInfo : InvalidData: (:) [Save-HTMLReport], ParameterBindingValidationException
+ FullyQualifiedErrorId : ParameterArgumentValidationErrorNullNotAllowed,Save-HTMLReport
On the same server do you get a error if you try and run: Get-ADComputer -Filter * -Properties *
No i don’t
It works fine and does give me the list of computers. Even i copied that line and executed it manually and have got proper result but with this full script it gives me error and no html output no graphs.
Amazing stuff!
can you also integrate it for services like Sharepoint, Teams as well!
I love this report. Is there any plans to update it?
Hi Brad,
This was a great learning. Thank you for a great post.
May I ask a question?
I am trying to change font background color of a PScustomobject. How can I do this if I use this module?
For eaxmple I want the value “Connection error’ as shown below with d background color in my html report.
My code:
$obj= [PSCustomObject]@{
‘Name’ = $Name
‘Log’ = ‘Connection error’
}
Hi Brad,
A great work. Can you give me a new version with Microsoft Graph, please, because I’m newbie with Powershell.
Best regards.
Hi,
Can you tell me how I replace this old command with his parameter in Microsoft graph, please ?
$PrivAdmins = Get-AzureADDirectoryRoleMember -ObjectId $privadminrole.ObjectId
Thank u
Hi,
You can replace your command by:
Get-MgDirectoryRoleMember -DirectoryRoleId $privadminrole.Id