View and Export your Intune Device Management Scripts Using the Microsoft Graph PowerShell SDK
Table of Contents
Introduction
If you’re familiar with Intune, you’re likely aware of its capability to deploy platform or PowerShell scripts to your endpoint devices. Unfortunately, once you upload your script to the portal, there is (at the time of writing this) no easy way to view the script content or download it.
Resolution
To quickly and easily get Intune scripts, I created a function that allows me to export all the scripts from a tenant en masse and view them in the shell or terminal. Sometimes, I am auditing a new tenant and have no need to download the scripts to my local machine; I need to audit the code behind it to get an idea of what is being performed. Additionally, I added some other quality-of-life features that I did not find in other scripts online.
Features
Platform Agnostic
The function will work on PowerShell Core (pwsh) and Windows PowerShell. Allowing you to run this on MacOS, Linux, and Windows without issues.
Pagination
There are a lot of other scripts that have very similar functionality out there. One thing I noticed with most of them is that they have no logic to account for pagination. I believe that there are two possible reasons for this:
- By default, a single request may return a maximum of 100 items. So, if you have over 100 scripts in your Intune tenant, you must perform multiple API calls to gather them all. Many companies may never see 100 scripts in their tenant, so there is no reason to build in pagination logic.
- Other SDK cmdlets perform pagination for you automatically, so there is no reason to think about it.
Get-MgUser
andGet-MgGroup
has the parameter, “All
” which gets all results for you automatically.
However, the SDK does not have a built-in cmdlet to get Device Configuration scripts, so we must use Invoke-MGGraphRequest
which does not handle pagination on its own.
$Request = @{
Uri = $Uri
Method = "GET"
}
#Invoke the Microsoft Graph request
$data = Invoke-MGGraphRequest @Request
while ($data.'@odata.nextLink') {
$resultsArray += $data.value
$Request.Uri = $data.'@odata.nextLink'
$data = Invoke-MGGraphRequest @Request
# to avoid throttling, the loop will sleep for 3 seconds
Start-Sleep -Seconds 3
}
$resultsArray += $data.value
Directory Verification
Another feature I wanted to add was ensuring the directory where you specified to have the scripts exported was present. If it weren’t, it would create it automatically instead of just throwing an error.
#Test the export folder if the variable is not null and create it if it does not exist
if ($exportDirectory -and !(Test-Path -Path $exportDirectory)) {
Write-Verbose "Creating Export Folder"
$newDirectory = @{
Path = $exportDirectory
ItemType = "Directory"
Force = $true
ErrorAction = "Stop"
}
# Create the root export directory
New-Item @newDirectory | Out-Null
}
Quick View
The last feature was the ability to view the scripts within the shell quickly. Sometimes, I do not need to export the script to my local machine but may need to view its contents quickly to understand what it is doing. In the picture below, you can see that I first view a table of all my Intune scripts, then select the last script and view its contents.
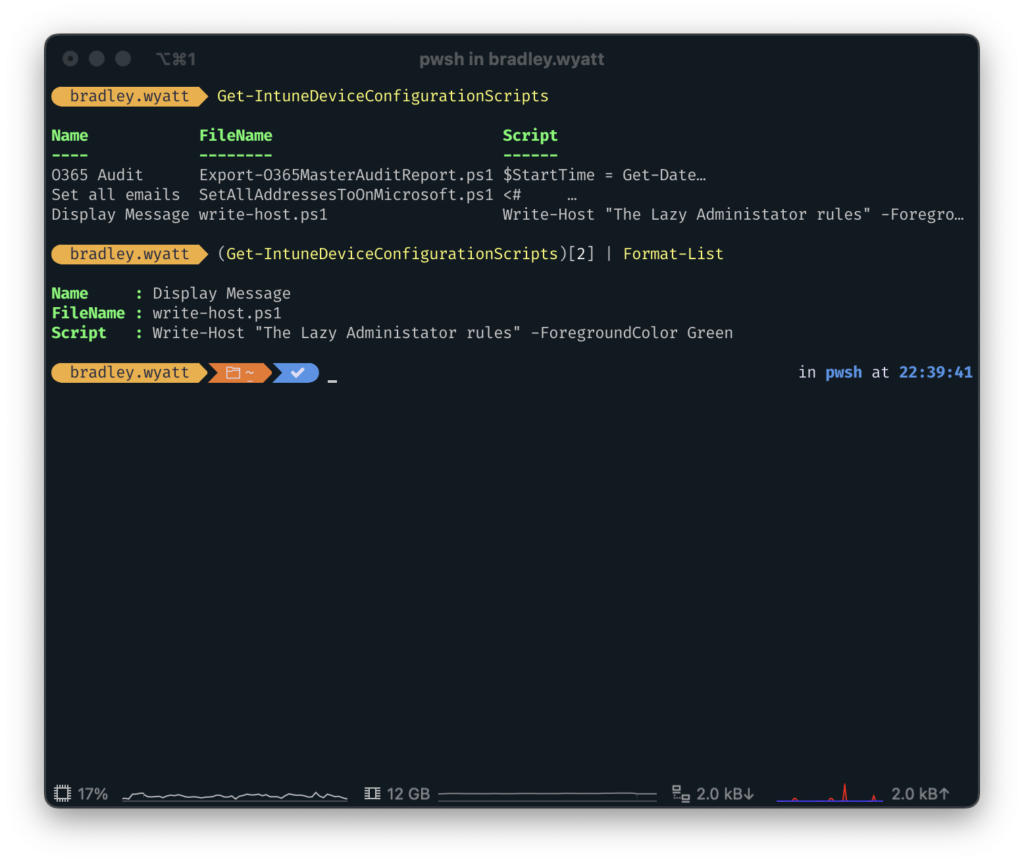
Even if the script spans multiple lines, you can still view it all in the shell.
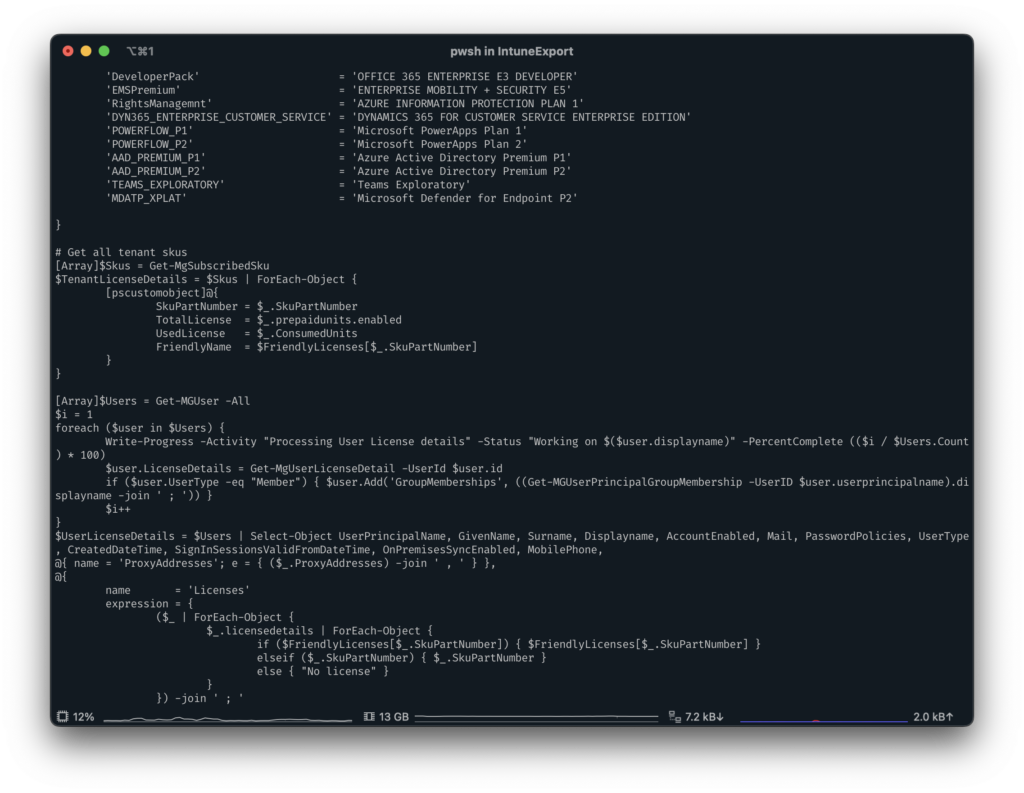
Folder Export
You can also choose to export all of the scripts to a specified directory. Doing so will create a subfolder with the name matching the script within the Intune portal.
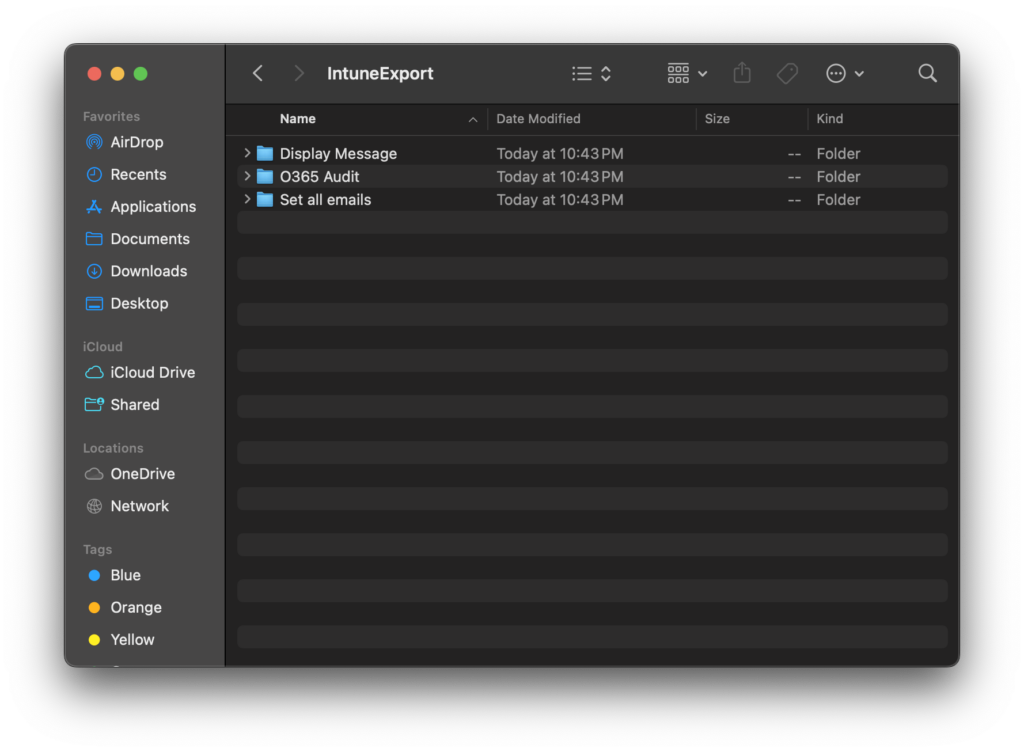
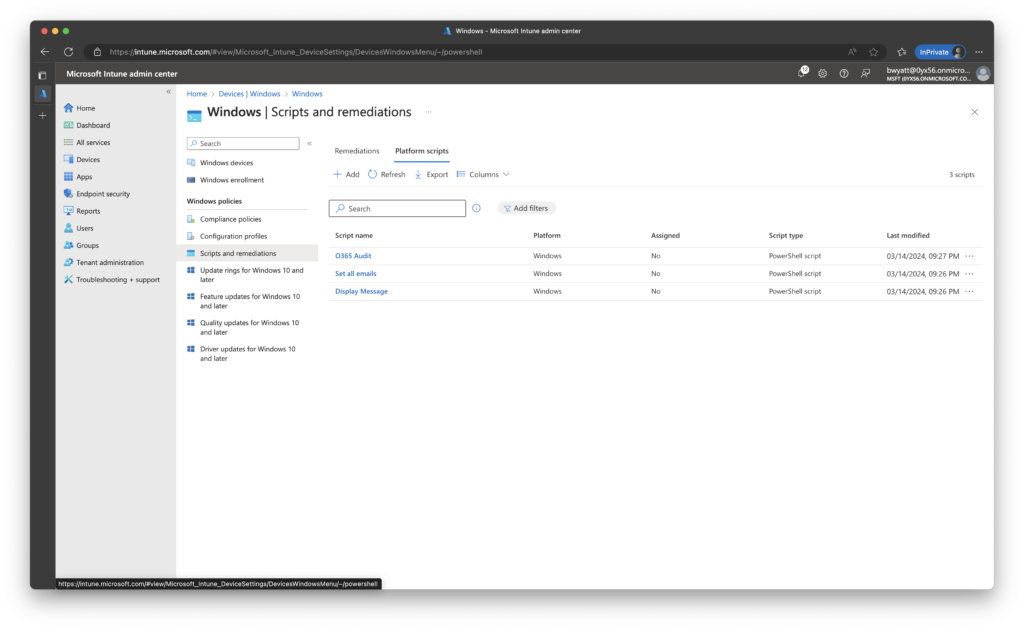
How To Run It
Note: The function requires you to have Microsoft.Graph.Authentication
module, which you can install by running Install-Module -Name Microsoft.Graph.Authentication
. The function will check for this module beforehand and attempt to install it if it’s not present.
- The first step is to download the code from GitHub.
- Next, copy and paste it into your terminal, dot-slash the script, add it to your PowerShell Profile, or run it in VSCode. For my example, I will just run it in VSCode.
- Once I have run it so the function is in memory, I can call the function by typing:
Get-IntuneDeviceConfigurationScripts
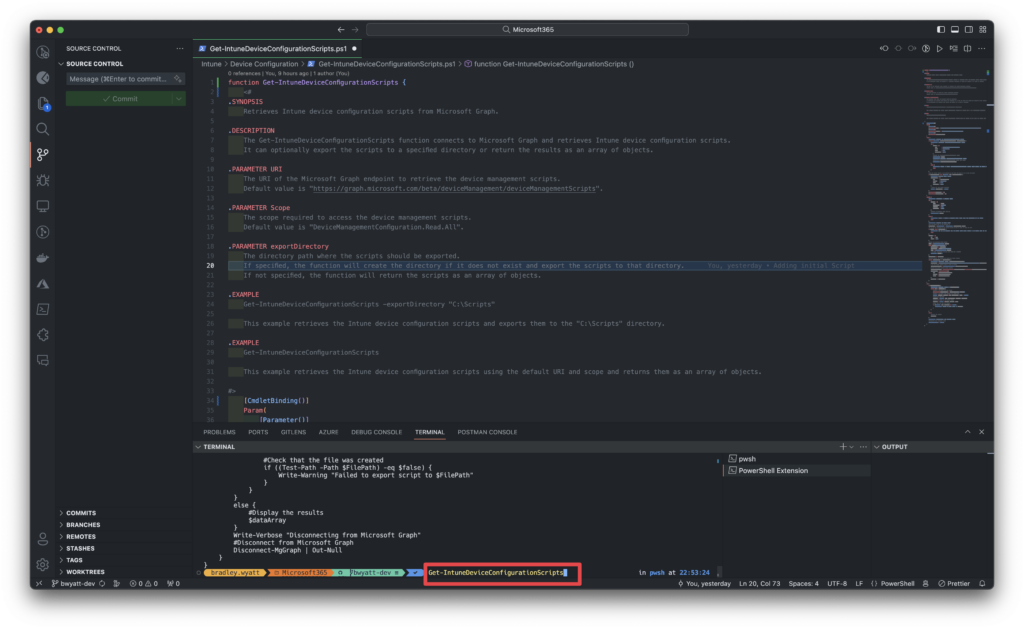
4. Next, I will be asked to authenticate and provide admin consent; you must be signed into the tenant with an account with the appropriate permissions, such as a Global Administrator. The function requires a single permission scope of DeviceManagementConfiguration.Read.All
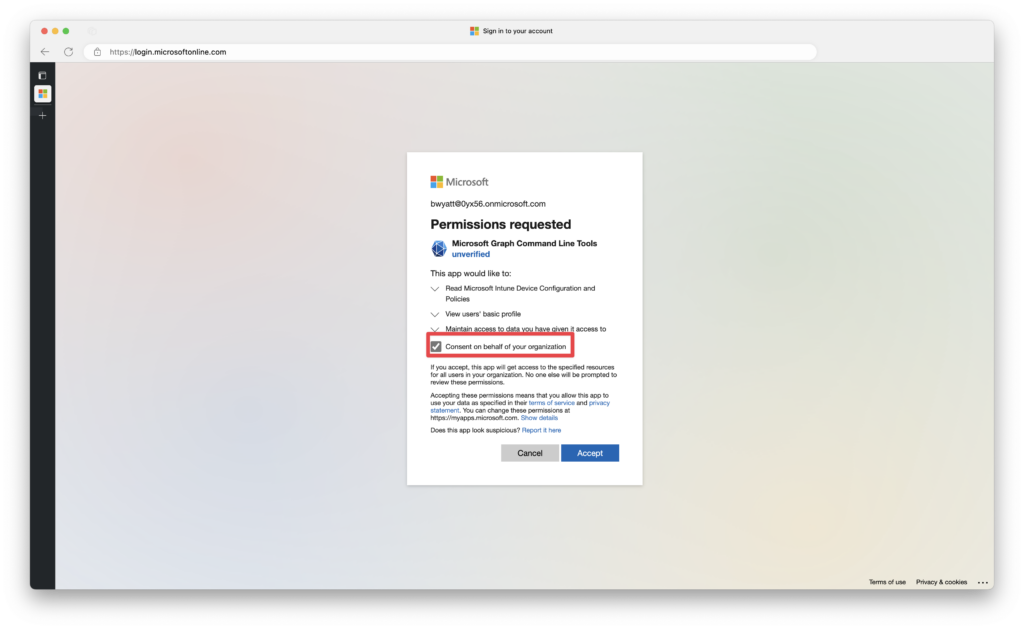
Parameters
The function has three parameters, two of which contain default values:
- Uri: Default value is the API endpoint to get device management scripts:
https://graph.microsoft.com/beta/deviceManagement/deviceManagementScripts
- Scope: The permission needed to get device management scripts; the default value is
DeviceConfiguration.Read.All
- exportDirectory (optional): The directory path to output the device management scripts
The following code will show you your scripts within the console:
Get-IntuneDeviceConfigurationScripts
The following code will export all of your scripts to a specific directory. If the directory is not present, it will automatically create it for you as long as it has permission:
Get-IntuneDeviceConfigurationScripts -exportDirectory "/users/bwyatt/Desktop/Output"
Get-IntuneDeviceConfigurationScripts -exportDirectory "C:\Temp"
Authentication Additional Information
Since we are using the PowerShell SDK to access the Mircosoft Graph API, we do not need to make our own service principal, but you may be wondering how we authenticate.
The PowerShell SDK uses the Microsoft Graph Command Line Tools service principal with an AppID of 14d82eec-204b-4c2f-b7e8-296a70dab67e
. You can view it in the Azure Portal by going to Entra ID > Enterprise Applications
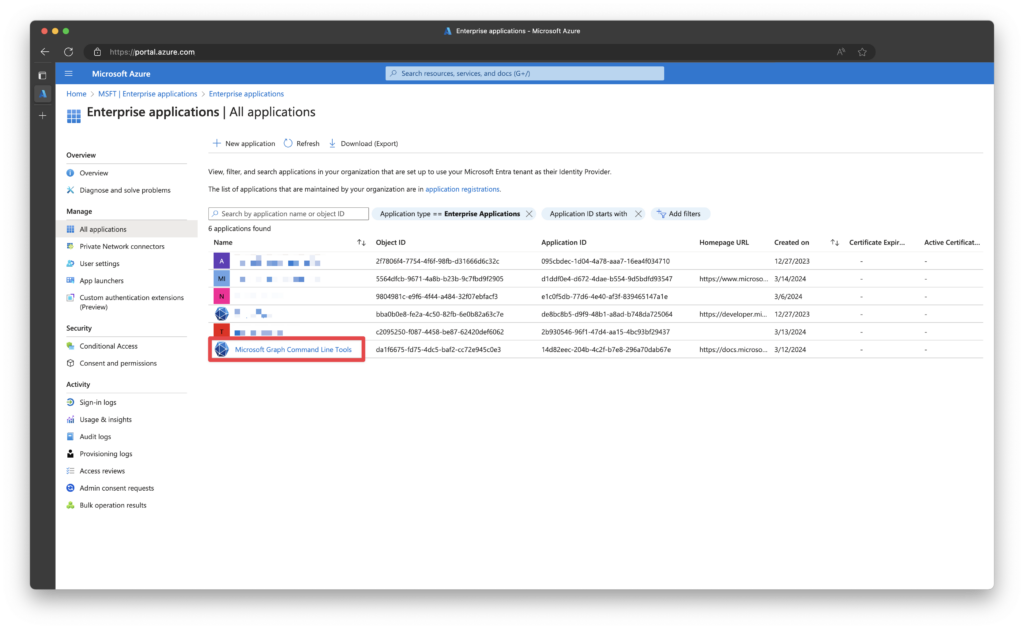
You can view all permissions assigned to the service principal in the Permissions section.
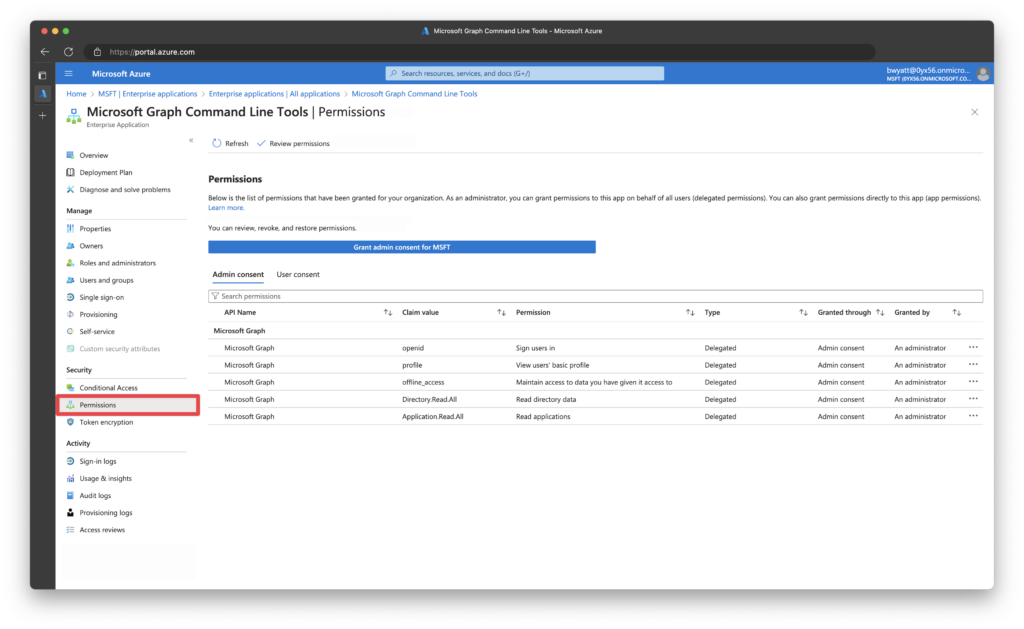
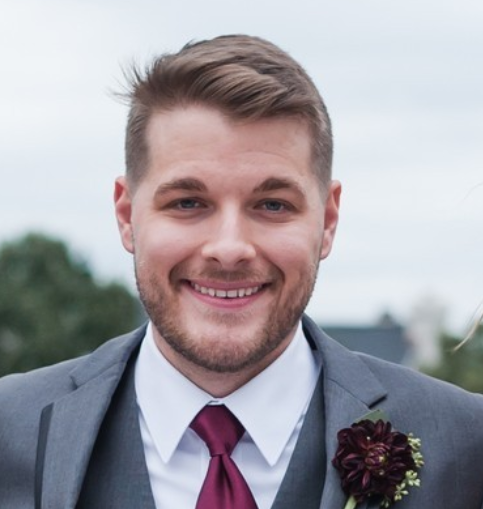
My name is Bradley Wyatt; I am a 5x Microsoft Most Valuable Professional (MVP) in Microsoft Azure and Microsoft 365. I have given talks at many different conferences, user groups, and companies throughout the United States, ranging from PowerShell to DevOps Security best practices, and I am the 2022 North American Outstanding Contribution to the Microsoft Community winner.